#go
Rulesets (26)
Scan code for potential security issues that require additional review. Recommended for teams looking to set up guardrails or to flag troublesome spots for further review.
Default ruleset for Go, curated by Semgrep.
Use Semgrep as a universal linter to identify vulnerabilities in your code base with the gosec (https://github.com/securego/gosec) rule pack.
Scan code for potential security issues that require additional review. Recommended for teams looking to set up guardrails or to flag troublesome spots for further review.

Secure defaults for XSS in Go.
A collection of opinionated rules for best practices in popular languages. Recommended for users who want really strict coding standards.

Secure defaults for XSS prevention
Find common bugs, errors, and logic issues in popular languages.
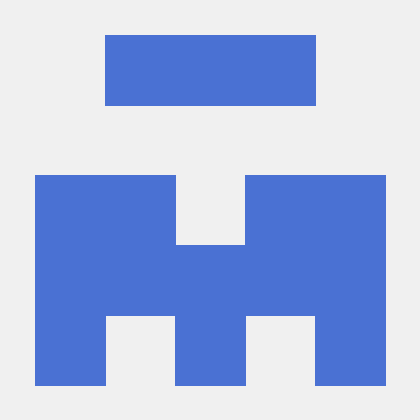
Ensure your code communicates over encrypted channels instead of plaintext.
Rules for detecting secrets checked into version control

Ruleset accompanying Semgrep OWASP presentation.
Use recommended rulesets specific to your project. Auto config is not a ruleset but a mode that scans for languages and frameworks and then uses the Semgrep Registry to select recommended rules. Semgrep will send a list of languages, frameworks, and your project URL to the Registry when using auto mode (but code is never uploaded).
Written by the Trail of Bits security experts. See https://github.com/trailofbits/semgrep-rules for more.

Omni pack for insecure transport rules

Secure defaults for Command injection prevention
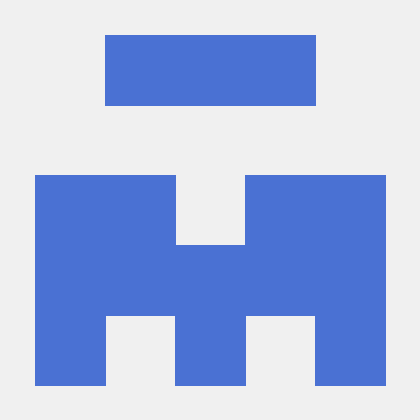
Ensure your code communicates over encrypted channels instead of plaintext.

Scan for runtime errors, logic bus, and high-confidence security vulnerabilities. Recommended for use in CI to block serious issues from reaching production. Supports Python, Java, JavaScript, and Go.

Scan for runtime errors, logic bus, and high-confidence security vulnerabilities. Recommended for use in CI to block serious issues from reaching production.
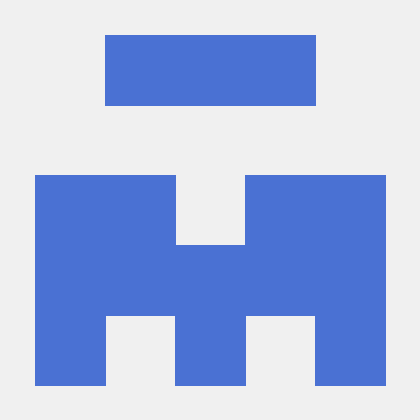
SQL injection guardrails. Checks for non-constant SQL queries and other SQLi.

Ruleset for OWASP SF
This is a placeholder for Semgrep Secrets
Rules for finding odd Go code. See github.com/dgryski/semgrep-go to contribute.
This rulepack powers the Semgrep Secrets product https://semgrep.dev/products/semgrep-secrets. If you are interested in trialing Semgrep Secrets reach out to sales@semgrep.com.
Rules (234)

Odd use of anonymous structs for function arguments

An HTTP redirect was found to be crafted from user-input `$REQUEST`. This can lead to open redirect vulnerabilities, potentially allowing attackers to redirect users to malicious web sites. It is recommend where possible to not allow user-input to craft the redirect URL. When user-input is necessary to craft the request, it is recommended to follow OWASP best practices to restrict the URL to domains in an allowlist.
Function bar detected

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

TODO in error handling code

odd hash.Sum call flow

calling json.Encode() on an http.ResponseWriter will set Content-Type text/plain

MarshalJSON with a pointer receiver has surprising results: https://github.com/golang/go/issues/22967

Try using math/bits instead

missing new relic end transaction

Odd bitwise expression

Odd compound += or -= expression

Odd bits.LeadingZeros() expression should perhaps be bits.Len()

64-bit integer parsed and downcast to u/int32

io.ReadFull() returns err == nil iff n == len(slice)

return nil instead of nil value

unless checking for wall clock inconsistencies, use !$T1.After($T2)

unless checking for wall clock inconsistencies, use !$T1.Before($T2)

use net.JoinHostPort instead of fmt.Sprintf($XX, $NET)

did you want path.Join() or filepath.Join()?

Odd sequence of ifs

Detected MD5 hash algorithm which is considered insecure. MD5 is not collision resistant and is therefore not suitable as a cryptographic signature. Use SHA256 or SHA3 instead.

Detected SHA1 hash algorithm which is considered insecure. SHA1 is not collision resistant and is therefore not suitable as a cryptographic signature. Use SHA256 or SHA3 instead.

use fmt.Fprintf($W, $...VALS) instead of fmt.Sprintf and []byte conversion

Detected a possible denial-of-service via a zip bomb attack. By limiting the max bytes read, you can mitigate this attack. `io.CopyN()` can specify a size.

Missing mutex unlock (`$T` variable) before returning from a function. This could result in panics resulting from double lock operations

Read() can return n bytes and io.EOF

ctx.Done() and time.After/time.NewTicker

passing an http-request scoped Context to a goroutine

return nil err instead of nil value

Odd comparison

Odd comparison

Odd comparison

Odd comparison

Odd comparison

Odd comparison

Odd comparison

Odd comparison

ioutil.NopCloser is deprecated

ioutil.ReadAll is deprecated

ioutil.ReadDir is deprecated

ioutil.ReadFile is deprecated

ioutil.TempDir is deprecated

ioutil.TempFile is deprecated

ioutil.WriteFile is deprecated

ioutil.Discard is deprecated

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

use net/mail Address.String() instead of fmt.Sprintf()

This code contains bidirectional (bidi) characters. While this is useful for support of right-to-left languages such as Arabic or Hebrew, it can also be used to trick language parsers into executing code in a manner that is different from how it is displayed in code editing and review tools. If this is not what you were expecting, please review this code in an editor that can reveal hidden Unicode characters.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

A session cookie was detected without setting the 'Secure' flag. The 'secure' flag for cookies prevents the client from transmitting the cookie over insecure channels such as HTTP. Set the 'Secure' flag by setting 'Secure' to 'true' in the Options struct.

Missing `RUnlock` on an `RWMutex` (`$T` variable) lock before returning from a function

Downcasting or changing sign of an integer with `$CAST_METHOD` method

A `sync.Mutex` is copied in function `$FUNC` given that `$T` is value receiver. As a result, the struct `$T` may not be locked as intended

The function is vulnerable to DLL hijacking attacks. Use `windows.NewLazySystemDLL()` function to limit DLL search to the Windows directory

Writing `$MAP` from multiple goroutines is not concurrency safe

Converting RemoteAddr to net.IP is probably wrong

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.
Url provided to HTTP request as taint input

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

it is good practice to call context cancellation function, $X(), in any case

Calling `$WG.Add` inside of an anonymous goroutine may result in `$WG.Wait` waiting for more or less calls to `$WG.Done()` than expected

Calling `$WG.Wait()` inside a loop blocks the call to `$WG.Done()`

Misspelling of MarshalJSON.

Misspelling of UnmarshalJSON.

Misspelling of MarshalYAML.

Misspelling of UnmarshalYAML.

Iteration over a possibly empty map `$C`. This is likely a bug or redundant code

use $W.Write($VAR) instead of fmt.Fprint when $VAR is []byte

Bad nil guard

Caret (^) is not exponentiation

QueryxContext rows must be closed (or use ExecContext)

Consider to use well-defined context

Use errors.Is($ERR, net.ErrClosed) instead

superfluous nil err check before return

Comparing a MAC with bytes.Equal()

calling hmac.New with unchanging hash.New

Maybe bad sort.Slice() less function

Use err.Error() instead

use $W.Write($VAR) instead of io.WriteString when $VAR is []byte

maybe returning wrong error

Wrong lock/unlock pair?

old-style go-fuzz fuzz function found

Detected a channel guarded with a mutex. Channels already have an internal mutex, so this is unnecessary. Remove the mutex. See https://hackmongo.com/page/golang-antipatterns/#guarded-channel for more information.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

New code should use errors.Is with the appropriate error type

New code should use errors.Is with the appropriate error type

New code should use errors.Is with the appropriate error type

New code should use errors.Is with the appropriate error type

Leaky use of time.After in for-select, see: https://groups.google.com/g/golang-nuts/c/cCdm0Ixwi9A/m/jMiJJScAEAAJ

Potential goroutine leak due to unbuffered channel send inside loop or unbuffered channel receive in select block

Potential `$FOO` nil dereference when `$BAR` is called

The `func ($O *$CODEC) ReadRequestBody($ARG $TYPE) error` function does not handle `nil` argument, as the `ServerCodec` interface requires. An incorrect implementation could lead to denial of service

Appending `$SLICE` from multiple goroutines is not concurrency safe

Detected SQL statement that is tainted by `$EVENT` object. This could lead to SQL injection if the variable is user-controlled and not properly sanitized. In order to prevent SQL injection, use parameterized queries or prepared statements instead. You can use prepared statements with the 'Prepare' and 'PrepareContext' calls.

Detected user input used to manually construct a SQL string. This is usually bad practice because manual construction could accidentally result in a SQL injection. An attacker could use a SQL injection to steal or modify contents of the database. Instead, use a parameterized query which is available by default in most database engines. Alternatively, consider using an object-relational mapper (ORM) such as Sequelize which will protect your queries.

Variable $VAR is assigned from two different sources: '$Y' and '$R'. Make sure this is intended, as this could cause logic bugs if they are treated as they are the same object.

A session cookie was detected without setting the 'HttpOnly' flag. The 'HttpOnly' flag for cookies instructs the browser to forbid client-side scripts from reading the cookie which mitigates XSS attacks. Set the 'HttpOnly' flag by setting 'HttpOnly' to 'true' in the Options struct.

The Origin header in the HTTP WebSocket handshake is used to guarantee that the connection accepted by the WebSocket is from a trusted origin domain. Failure to enforce can lead to Cross Site Request Forgery (CSRF). As per "gorilla/websocket" documentation: "A CheckOrigin function should carefully validate the request origin to prevent cross-site request forgery."

Detected usage of dangerous method $METHOD which does not escape inputs (see link in references). If the argument is user-controlled, this can lead to SQL injection. When using $METHOD function, do not trust user-submitted data and only allow approved list of input (possibly, use an allowlist approach).

Found an insecure gRPC connection using 'grpc.WithInsecure()'. This creates a connection without encryption to a gRPC server. A malicious attacker could tamper with the gRPC message, which could compromise the machine. Instead, establish a secure connection with an SSL certificate using the 'grpc.WithTransportCredentials()' function. You can create a create credentials using a 'tls.Config{}' struct with 'credentials.NewTLS()'. The final fix looks like this: 'grpc.WithTransportCredentials(credentials.NewTLS(<config>))'.

Found an insecure gRPC server without 'grpc.Creds()' or options with credentials. This allows for a connection without encryption to this server. A malicious attacker could tamper with the gRPC message, which could compromise the machine. Include credentials derived from an SSL certificate in order to create a secure gRPC connection. You can create credentials using 'credentials.NewServerTLSFromFile("cert.pem", "cert.key")'.

Detected the decoding of a JWT token without a verify step. Don't use `ParseUnverified` unless you know what you're doing This method parses the token but doesn't validate the signature. It's only ever useful in cases where you know the signature is valid (because it has been checked previously in the stack) and you want to extract values from it.

A hard-coded credential was detected. It is not recommended to store credentials in source-code, as this risks secrets being leaked and used by either an internal or external malicious adversary. It is recommended to use environment variables to securely provide credentials or retrieve credentials from a secure vault or HSM (Hardware Security Module).

Detected a hidden goroutine. Function invocations are expected to synchronous, and this function will execute asynchronously because all it does is call a goroutine. Instead, remove the internal goroutine and call the function using 'go'.

`$VALUE` is a loop pointer that may be exported from the loop. This pointer is shared between loop iterations, so the exported reference will always point to the last loop value, which is likely unintentional. To fix, copy the pointer to a new pointer within the loop.

Detected conversion of the result of a strconv.Atoi command to an int16. This could lead to an integer overflow, which could possibly result in unexpected behavior and even privilege escalation. Instead, use `strconv.ParseInt`.

Detected conversion of the result of a strconv.Atoi command to an int32. This could lead to an integer overflow, which could possibly result in unexpected behavior and even privilege escalation. Instead, use `strconv.ParseInt`.

Detected file permissions that are set to more than `0600` (user/owner can read and write). Setting file permissions to higher than `0600` is most likely unnecessary and violates the principle of least privilege. Instead, set permissions to be `0600` or less for os.Chmod, os.Mkdir, os.OpenFile, os.MkdirAll, and ioutil.WriteFile

`path.Join(...)` always joins using a forward slash. This may cause issues on Windows or other systems using a different delimiter. Use `filepath.Join(...)` instead which uses OS-specific path separators.

Detected useless comparison operation `$X == $X` or `$X != $X`. This will always return 'True' or 'False' and therefore is not necessary. Instead, remove this comparison operation or use another comparison expression that is not deterministic.

Detected useless if statement. 'if (True)' and 'if (False)' always result in the same behavior, and therefore is not necessary in the code. Remove the 'if (False)' expression completely or just the 'if (True)' comparison depending on which expression is in the code.

Detected an if block that checks for the same condition on both branches (`$X`). The second condition check is useless as it is the same as the first, and therefore can be removed from the code,

Detected identical statements in the if body and the else body of an if-statement. This will lead to the same code being executed no matter what the if-expression evaluates to. Instead, remove the if statement.

The package `net/http/cgi` is on the import blocklist. The package is vulnerable to httpoxy attacks (CVE-2015-5386). It is recommended to use `net/http` or a web framework to build a web application instead.

Disabled host key verification detected. This allows man-in-the-middle attacks. Use the 'golang.org/x/crypto/ssh/knownhosts' package to do host key verification. See https://skarlso.github.io/2019/02/17/go-ssh-with-host-key-verification/ to learn more about the problem and how to fix it.

Do not use `math/rand`. Use `crypto/rand` instead.

`MinVersion` is missing from this TLS configuration. By default, TLS 1.2 is currently used as the minimum when acting as a client, and TLS 1.0 when acting as a server. General purpose web applications should default to TLS 1.3 with all other protocols disabled. Only where it is known that a web server must support legacy clients with unsupported an insecure browsers (such as Internet Explorer 10), it may be necessary to enable TLS 1.0 to provide support. Add `MinVersion: tls.VersionTLS13' to the TLS configuration to bump the minimum version to TLS 1.3.

SSLv3 is insecure because it has known vulnerabilities. Starting with go1.14, SSLv3 will be removed. Instead, use 'tls.VersionTLS13'.

Detected an insecure CipherSuite via the 'tls' module. This suite is considered weak. Use the function 'tls.CipherSuites()' to get a list of good cipher suites. See https://golang.org/pkg/crypto/tls/#InsecureCipherSuites for why and what other cipher suites to use.

Detected use of the 'none' algorithm in a JWT token. The 'none' algorithm assumes the integrity of the token has already been verified. This would allow a malicious actor to forge a JWT token that will automatically be verified. Do not explicitly use the 'none' algorithm. Instead, use an algorithm such as 'HS256'.

Detected DES cipher algorithm which is insecure. The algorithm is considered weak and has been deprecated. Use AES instead.

Detected RC4 cipher algorithm which is insecure. The algorithm has many known vulnerabilities. Use AES instead.

RSA keys should be at least 2048 bits

Detected non-static command inside Write. Audit the input to '$CW.Write'. If unverified user data can reach this call site, this is a code injection vulnerability. A malicious actor can inject a malicious script to execute arbitrary code.

Detected non-static command inside exec.Cmd. Audit the input to 'exec.Cmd'. If unverified user data can reach this call site, this is a code injection vulnerability. A malicious actor can inject a malicious script to execute arbitrary code.

Detected non-static command inside Command. Audit the input to 'exec.Command'. If unverified user data can reach this call site, this is a code injection vulnerability. A malicious actor can inject a malicious script to execute arbitrary code.

Detected non-static command inside Exec. Audit the input to 'syscall.Exec'. If unverified user data can reach this call site, this is a code injection vulnerability. A malicious actor can inject a malicious script to execute arbitrary code.

It looks like MD5 is used as a password hash. MD5 is not considered a secure password hash because it can be cracked by an attacker in a short amount of time. Use a suitable password hashing function such as bcrypt. You can use the `golang.org/x/crypto/bcrypt` package.

Detected a network listener listening on 0.0.0.0 or an empty string. This could unexpectedly expose the server publicly as it binds to all available interfaces. Instead, specify another IP address that is not 0.0.0.0 nor the empty string.

A session cookie was detected without setting the 'HttpOnly' flag. The 'HttpOnly' flag for cookies instructs the browser to forbid client-side scripts from reading the cookie which mitigates XSS attacks. Set the 'HttpOnly' flag by setting 'HttpOnly' to 'true' in the Cookie.

A session cookie was detected without setting the 'Secure' flag. The 'secure' flag for cookies prevents the client from transmitting the cookie over insecure channels such as HTTP. Set the 'Secure' flag by setting 'Secure' to 'true' in the Options struct.

Detected a potentially dynamic ClientTrace. This occurred because semgrep could not find a static definition for '$TRACE'. Dynamic ClientTraces are dangerous because they deserialize function code to run when certain Request events occur, which could lead to code being run without your knowledge. Ensure that your ClientTrace is statically defined.

Found a formatted template string passed to 'template.HTML()'. 'template.HTML()' does not escape contents. Be absolutely sure there is no user-controlled data in this template. If user data can reach this template, you may have a XSS vulnerability.

Detected usage of 'http.FileServer' as handler: this allows directory listing and an attacker could navigate through directories looking for sensitive files. Be sure to disable directory listing or restrict access to specific directories/files.

The profiling 'pprof' endpoint is automatically exposed on /debug/pprof. This could leak information about the server. Instead, use `import "net/http/pprof"`. See https://www.farsightsecurity.com/blog/txt-record/go-remote-profiling-20161028/ for more information and mitigation.

Found a formatted template string passed to 'template. HTMLAttr()'. 'template.HTMLAttr()' does not escape contents. Be absolutely sure there is no user-controlled data in this template or validate and sanitize the data before passing it into the template.

String-formatted SQL query detected. This could lead to SQL injection if the string is not sanitized properly. Audit this call to ensure the SQL is not manipulable by external data.

Found a formatted template string passed to 'template.JS()'. 'template.JS()' does not escape contents. Be absolutely sure there is no user-controlled data in this template.

Found a formatted template string passed to 'template.URL()'. 'template.URL()' does not escape contents, and this could result in XSS (cross-site scripting) and therefore confidential data being stolen. Sanitize data coming into this function or make sure that no user-controlled input is coming into the function.

Found an HTTP server without TLS. Use 'http.ListenAndServeTLS' instead. See https://golang.org/pkg/net/http/#ListenAndServeTLS for more information.

Found data going from url query parameters into formatted data written to ResponseWriter. This could be XSS and should not be done. If you must do this, ensure your data is sanitized or escaped.

'reflect.MakeFunc' detected. This will sidestep protections that are normally afforded by Go's type system. Audit this call and be sure that user input cannot be used to affect the code generated by MakeFunc; otherwise, you will have a serious security vulnerability.

Detected string concatenation with a non-literal variable in a "database/sql" Go SQL statement. This could lead to SQL injection if the variable is user-controlled and not properly sanitized. In order to prevent SQL injection, use parameterized queries or prepared statements instead. You can use prepared statements with the 'Prepare' and 'PrepareContext' calls.

Detected string concatenation with a non-literal variable in a go-pg ORM SQL statement. This could lead to SQL injection if the variable is user-controlled and not properly sanitized. In order to prevent SQL injection, do not use strings concatenated with user-controlled input. Instead, use parameterized statements.

Detected string concatenation with a non-literal variable in a go-pg SQL statement. This could lead to SQL injection if the variable is user-controlled and not properly sanitized. In order to prevent SQL injection, use parameterized queries instead of string concatenation. You can use parameterized queries like so: '(SELECT ? FROM table, data1)'

Detected string concatenation with a non-literal variable in a pgx Go SQL statement. This could lead to SQL injection if the variable is user-controlled and not properly sanitized. In order to prevent SQL injection, use parameterized queries instead. You can use parameterized queries like so: (`SELECT $1 FROM table`, `data1)

If an attacker can supply values that the application then uses to determine which method or field to invoke, the potential exists for the attacker to create control flow paths through the application that were not intended by the application developers. This attack vector may allow the attacker to bypass authentication or access control checks or otherwise cause the application to behave in an unexpected manner.

Using the unsafe package in Go gives you low-level memory management and many of the strengths of the C language, but also steps around the type safety of Go and can lead to buffer overflows and possible arbitrary code execution by an attacker. Only use this package if you absolutely know what you're doing.

When working with web applications that involve rendering user-generated content, it's important to properly escape any HTML content to prevent Cross-Site Scripting (XSS) attacks. In Go, the `text/template` package does not automatically escape HTML content, which can leave your application vulnerable to these types of attacks. To mitigate this risk, it's recommended to use the `html/template` package instead, which provides built-in functionality for HTML escaping. By using `html/template` to render your HTML content, you can help to ensure that your web application is more secure and less susceptible to XSS vulnerabilities.

Detected directly writing or similar in 'http.ResponseWriter.write()'. This bypasses HTML escaping that prevents cross-site scripting vulnerabilities. Instead, use the 'html/template' package and render data using 'template.Execute()'.

Detected 'Fprintf' or similar writing to 'http.ResponseWriter'. This bypasses HTML escaping that prevents cross-site scripting vulnerabilities. Instead, use the 'html/template' package to render data to users.

Detected 'io.WriteString()' writing directly to 'http.ResponseWriter'. This bypasses HTML escaping that prevents cross-site scripting vulnerabilities. Instead, use the 'html/template' package to render data to users.

Detected 'printf' or similar in 'http.ResponseWriter.write()'. This bypasses HTML escaping that prevents cross-site scripting vulnerabilities. Instead, use the 'html/template' package to render data to users.

Semgrep could not determine that the argument to 'template.HTML()' is a constant. 'template.HTML()' and similar does not escape contents. Be absolutely sure there is no user-controlled data in this template. If user data can reach this template, you may have a XSS vulnerability. Instead, do not use this function and use 'template.Execute()'.

Detected enabling of "XMLParseNoEnt", which allows parsing of external entities and can lead to XXE if user controlled data is parsed by the library. Instead, do not enable "XMLParseNoEnt" or be sure to adequately sanitize user-controlled data when it is being parsed by this library.

File creation in shared tmp directory without using ioutil.Tempfile

`Clean` is not intended to sanitize against path traversal attacks. This function is for finding the shortest path name equivalent to the given input. Using `Clean` to sanitize file reads may expose this application to path traversal attacks, where an attacker could access arbitrary files on the server. To fix this easily, write this: `filepath.FromSlash(path.Clean("/"+strings.Trim(req.URL.Path, "/")))` However, a better solution is using the `SecureJoin` function in the package `filepath-securejoin`. See https://pkg.go.dev/github.com/cyphar/filepath-securejoin#section-readme.

Detected user input flowing into a manually constructed HTML string. You may be accidentally bypassing secure methods of rendering HTML by manually constructing HTML and this could create a cross-site scripting vulnerability, which could let attackers steal sensitive user data. Use the `html/template` package which will safely render HTML instead, or inspect that the HTML is rendered safely.

User data flows into this manually-constructed SQL string. User data can be safely inserted into SQL strings using prepared statements or an object-relational mapper (ORM). Manually-constructed SQL strings is a possible indicator of SQL injection, which could let an attacker steal or manipulate data from the database. Instead, use prepared statements (`db.Query("SELECT * FROM t WHERE id = ?", id)`) or a safe library.

File traversal when extracting zip archive

Detected non-static script inside otto VM. Audit the input to 'VM.Run'. If unverified user data can reach this call site, this is a code injection vulnerability. A malicious actor can inject a malicious script to execute arbitrary code.

Checks for disabling of TLS/SSL certificate verification. This should only be used for debugging purposes because it leads to vulnerability to MTM attacks.

Detects creations of tls configuration objects with an insecure MinVersion of TLS. These protocols are deprecated due to POODLE, man in the middle attacks, and other vulnerabilities.

Checks for outgoing connections to ftp servers with the ftp package. FTP does not encrypt traffic, possibly leading to PII being sent plaintext over the network. Instead, connect via the SFTP protocol.

Checks for requests to http (unencrypted) sites using gorequest, a popular HTTP client library. This is dangerous because it could result in plaintext PII being passed around the network.

Checks for requests to http (unencrypted) sites using grequests, a popular HTTP client library. This is dangerous because it could result in plaintext PII being passed around the network.

Checks for requests sent via http.NewRequest to http:// URLS. This is dangerous because the server is attempting to connect to a website that does not encrypt traffic with TLS. Instead, send requests only to https:// URLS.

Checks for requests sent via http.$FUNC to http:// URLS. This is dangerous because the server is attempting to connect to a website that does not encrypt traffic with TLS. Instead, send requests only to https:// URLS.

Checks for requests to http (unencrypted) sites using gorequest, a popular HTTP client library. This is dangerous because it could result in plaintext PII being passed around the network.

Checks for attempts to connect to an insecure telnet server using the package telnet. This is bad because it can lead to man in the middle attacks.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

Insufficient permissions to view rule definition. This rule is only visible to logged in users. Log in to see this rule.

A request was found to be crafted from user-input `$REQUEST`. This can lead to Server-Side Request Forgery (SSRF) vulnerabilities, potentially exposing sensitive data. It is recommend where possible to not allow user-input to craft the base request, but to be treated as part of the path or query parameter. When user-input is necessary to craft the request, it is recommended to follow OWASP best practices to prevent abuse, including using an allowlist.

Variable `$X` is likely modified and later used on error. In some cases this could result in panics due to a nil dereference